ESP32 Wifi gateway for Bresser weather stations
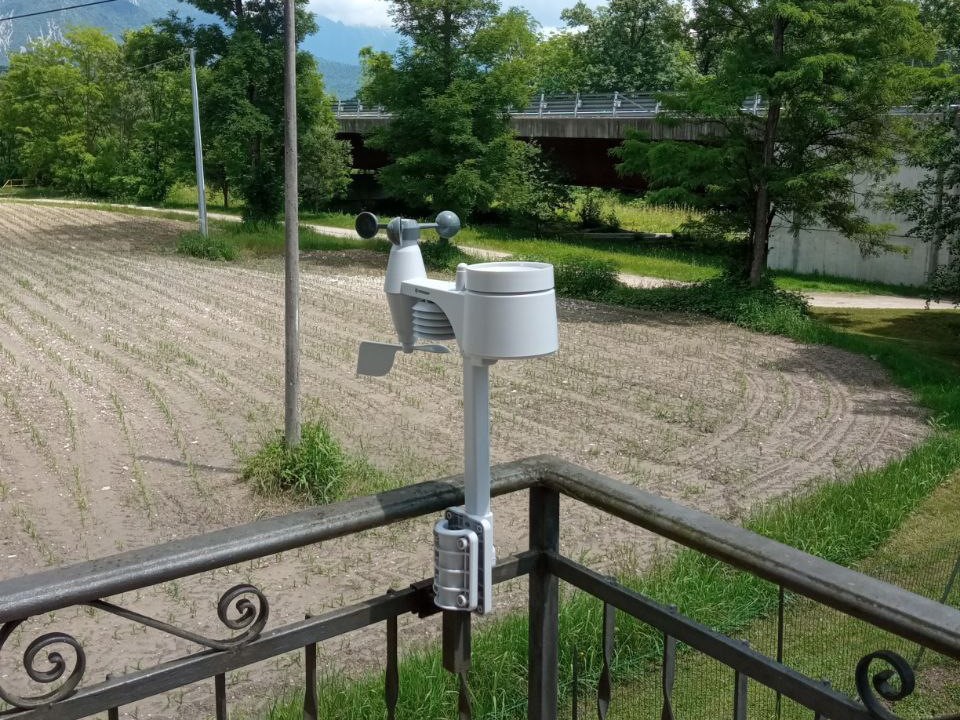
Index
- Introduction
- The problem
- Searching for a possible solution
- Prototype development
- Conclusions
- Future improvements
- Glossary
Introduction
Klinkon Electronics has been collaborating for years on many projects with DeLucaLabs, a maker lab near Sedico. Together with them we are developing a system to connect our environmental monitoring service AMMS (Autonomous Meteorological Monitoring System, a monitoring system with several interconnected weather stations for the purpose of acquiring data on environmental conditions and processing simple weather forecasts, creating an archive OpenData with the collected data) as many stations as possible, and to cut costs and technical difficulties we also looked for weather stations that could be easily modified, with a focus on cheap ones from Amazon, in this case the Bresser 5 in 1and with this article we want to explain how you can replicate the system we developed at home with just a few electronic components.
The problem
The station Bresser 5 in 1 consists of two units:
- Weather station with temperature, humidity, pressure, wind speed and direction and precipitation sensors
- Remote control display
These two units communicate with each other via radio on the free frequency 868 MHz.
Since it was therefore not possible to read the station data directly via the Internet, it was necessary to devise a solution for sending them to the AMMS project's central server for collecting and consulting meteorological data, which would allow via a Gateway to receive data sent by the weather station via radio frequencies and transmit it in turn via the Internet to the central server
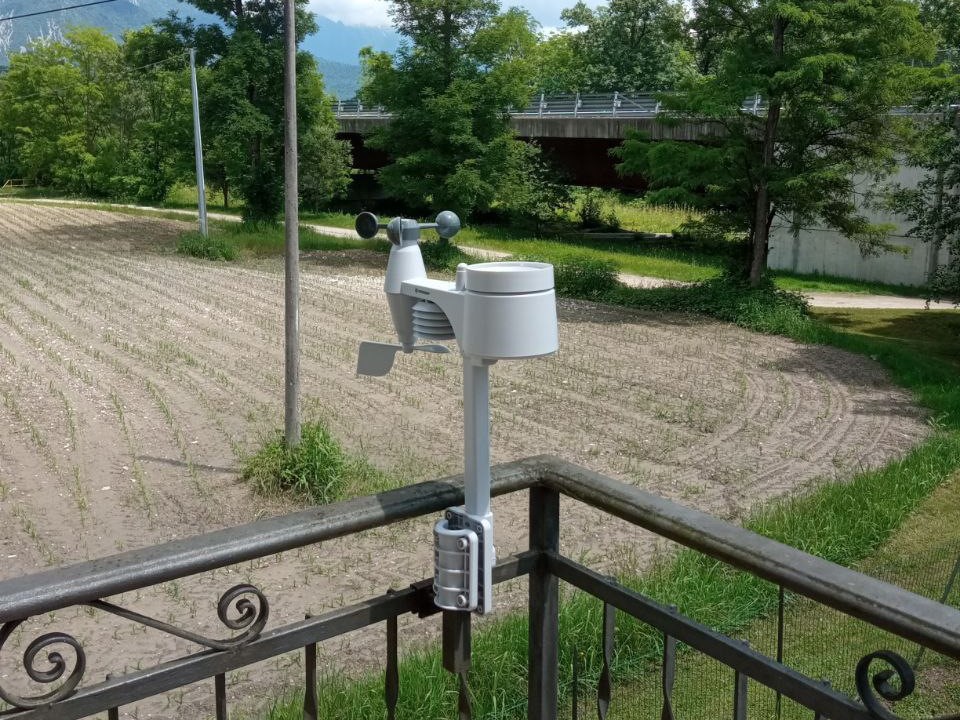
Searching for a possible solution
It is therefore necessary to develop a device that allows the data generated by the Bresser to be read and 'intercepted' as it is sent to the remote control display, while maintaining the stability of the connection over time and also consuming as little power as possible, as it will have to remain connected to the power supply and the Internet 24 hours a day.
Our hardwarists Lorenzo and Leonardo, after hours of research into possible solutions, decided to develop a Gateway IOTtaking a cue from the OpenSource BresserWeatherSensorReceiver by Matthias Prinkeconsisting of a radio module RFM95W-915S2 and driven by an ESP32, which will analyse the data received from the weather station and then send it via the Internet to our centralised system.
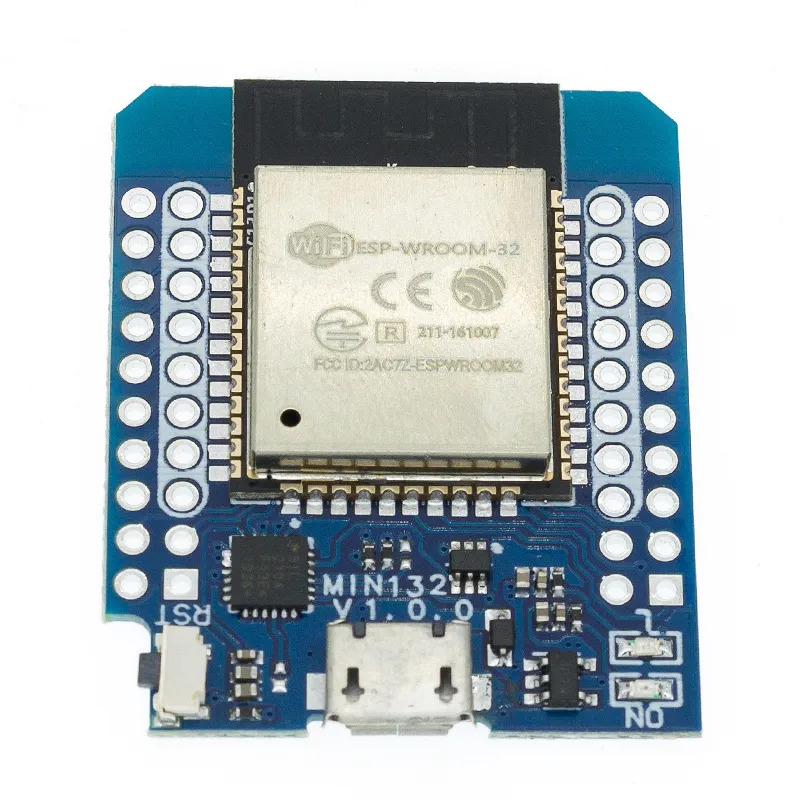
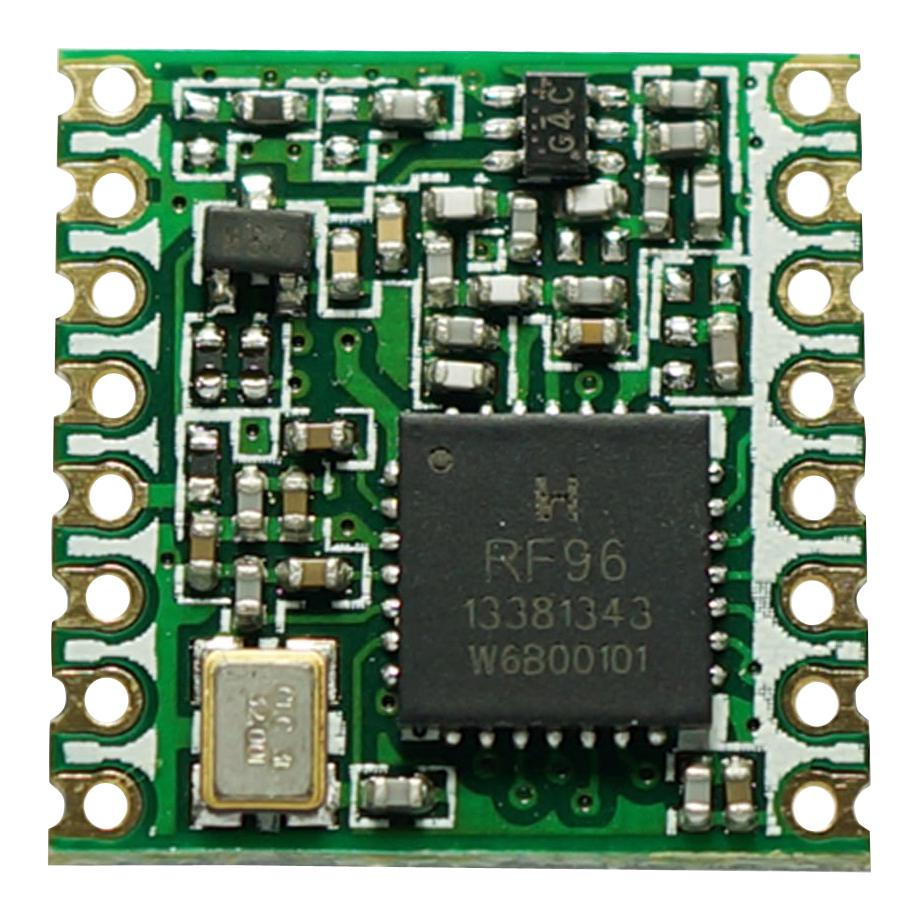
Prototype Development - Hardware
After searching for the components, we have ordered the parts and can finally create the prototype of the system, which you can also recreate by following these steps
Start by connecting the RF module to the WemosD1, following the table below:
RFM95W PIN | PIN ESP32 D1 Mini |
RESET | IO32 |
NSS | IO27 |
SCK | IO18 |
MOSI | IO23 |
MISO | IO19 |
GND | GND |
3.3V | 3.3V |
GOD0 | IO21 |
GOD1 | IO33 |
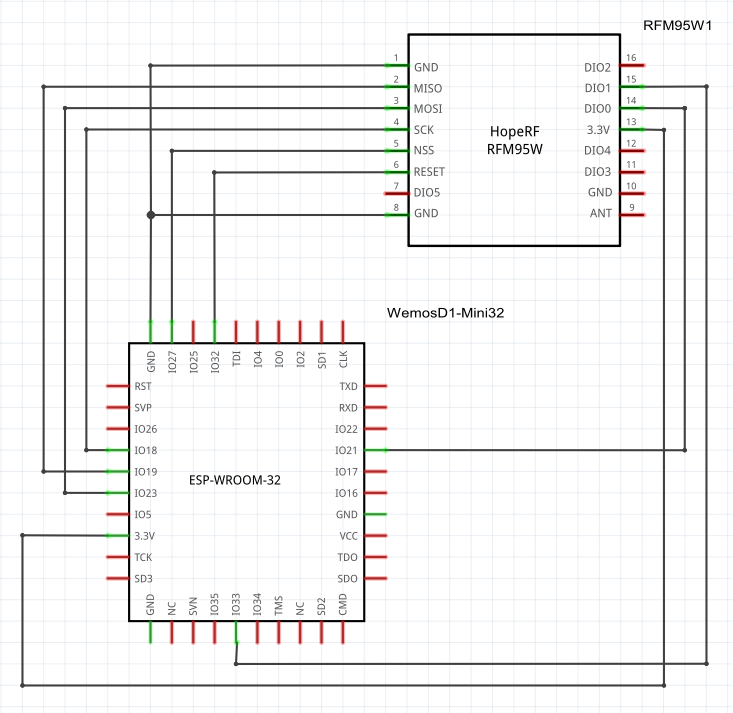
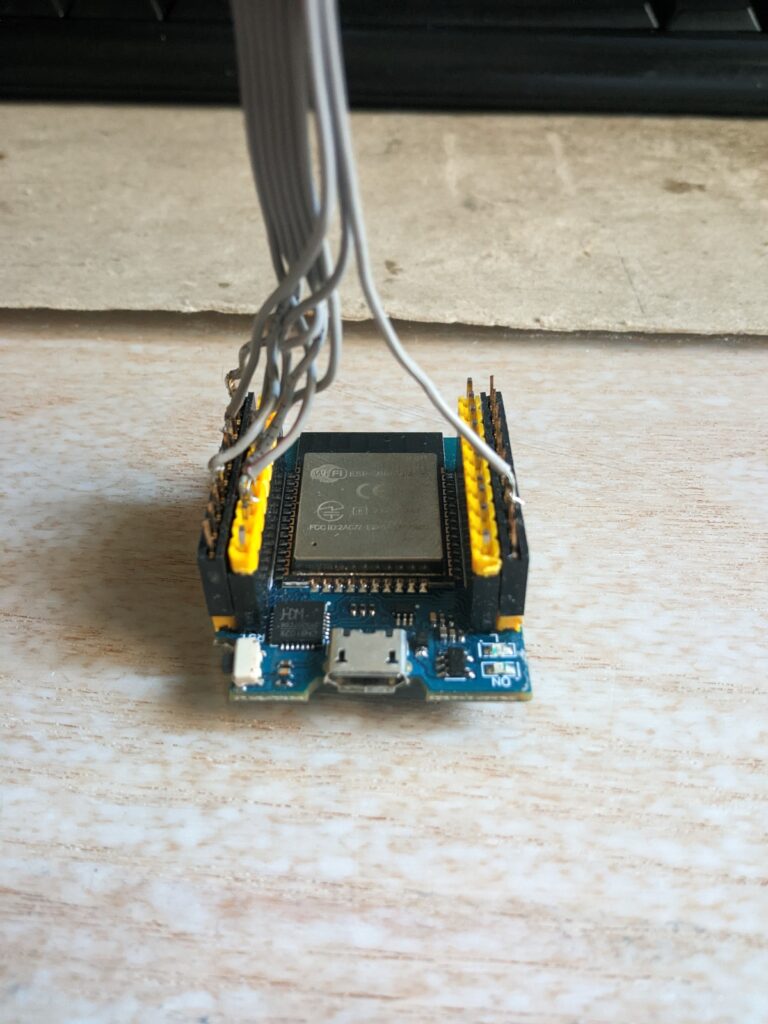
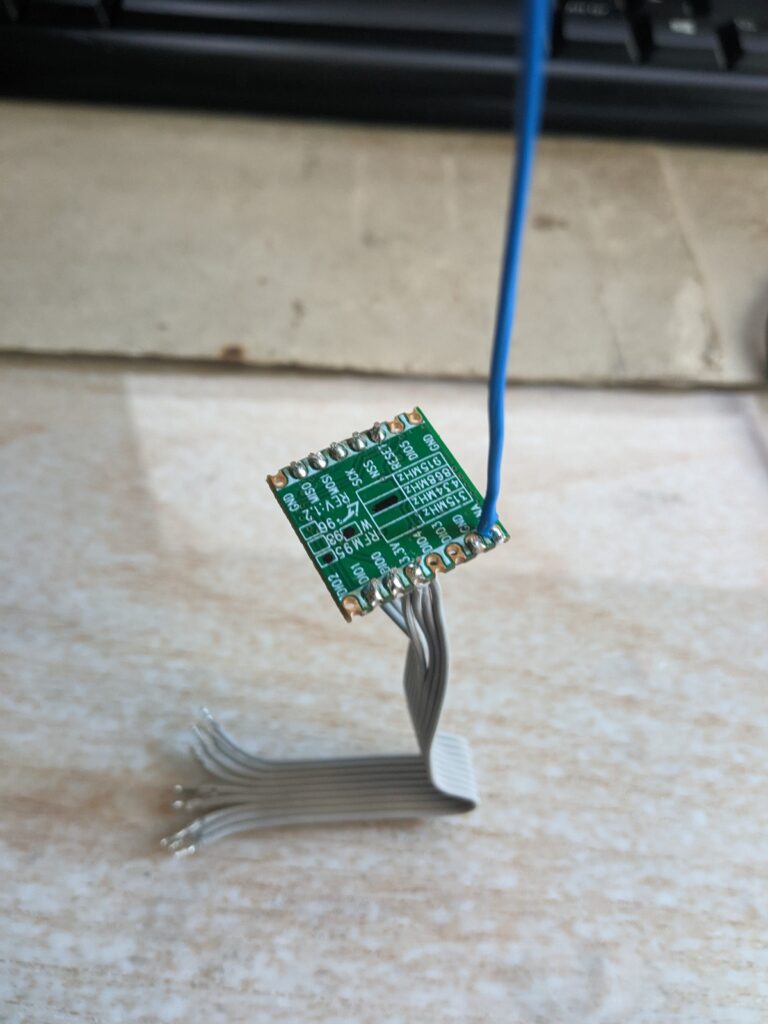
Although the setup is a bit tentative once the system is soldered and assembled like this, everything is ready to move on to the software part, the somewhat more complex part, which we will now explain in detail
*DISCLAIMER: The guide will explain how to interface the Bresser to send data to our AMMS weather system, with a token that you can request directly from us in our telegram group t.me/klinkonelectronicschathowever, the readings can also be sent via thingspeak with the addition of the corresponding library and are also visible via the serial monitor of the arduino IDE or VSCode
Prototype Development - Software
The Gateway's main programme is a fork of example sketches by Matthias Prinke with the addition of our library for sending meteorological data to the AMMS server.
It takes care of reading data from the RF module and uploading the data to the ESP serial and our AMMS system.
Below is the basic code that makes the system work. In case you are replicating our setup, simply download the original package from the GitHub at this linkopen it with PlatFormioIDE on VSCode and compile it after editing the relevant parts of code
#include <AMMSutils.h> #include <Arduino.h> #include <ArduinoJson.h> #include <WiFiManager.h> #include "WeatherSensorCfg.h" #include "WeatherSensor.h" String AMMS_TOKEN = "STATION_TOKEN_HERE"; WeatherSensor weatherSensor; WiFiManager wifiManager; AMMSutils amms; void onDisconnect(WiFiEvent_t event, WiFiEventInfo_t info) { wifiManager.autoConnect("Bresser Gateway"); } void setup() { Serial.begin(115200); Serial.setDebugOutput(true); Serial.println("Firmware version: 1.2"); weatherSensor.begin(); WiFi.mode(WIFI_STA); WiFi.onEvent(onDisconnect, WiFiEvent_t::ARDUINO_EVENT_WIFI_STA_DISCONNECTED); wifiManager.setDebugOutput(true); wifiManager.setConfigPortalTimeout(30); Serial.printf("Starting execution...\n"); wifiManager.autoConnect("Bresser Gateway"); amms.begin(AMMS_TOKEN); } void loop() { int const i = 0; weatherSensor.clearSlots(); int decode_status = weatherSensor.getMessage(); if (decode_status == DECODE_OK) { Serial.printf("Id: [%8X] Typ: [%X] Battery: [%s] ", weatherSensor.sensor[i].sensor_id, weatherSensor.sensor[i].s_type, weatherSensor.sensor[i].battery_ok ? "OK " : "Low"); if (weatherSensor.sensor[i].temp_ok) { Serial.printf("Temp: [%5.1fC] ", weatherSensor.sensor[i].temp_c); amms.setSensorField("temperature", weatherSensor.sensor[i].temp_c); } else { Serial.printf("Temp: [---.-C] "); } if (weatherSensor.sensor[i].humidity_ok) { Serial.printf("Hum: [%3d%%] ", weatherSensor.sensor[i].humidity); amms.setSensorField("humidity", weatherSensor.sensor[i].humidity); } else { Serial.printf("Hum: [---%%] "); } if (weatherSensor.sensor[i].wind_ok) { Serial.printf("Wind max: [%4.1fm/s] Wind avg: [%4.1fm/s] Wind dir: [%5.1fdeg] ", weatherSensor.sensor[i].wind_gust_meter_sec, weatherSensor.sensor[i].wind_avg_meter_sec, weatherSensor.sensor[i].wind_direction_deg); amms.setSensorField("wind_speed", weatherSensor.sensor[i].wind_avg_meter_sec); amms.setSensorField("wind_direction", weatherSensor.sensor[i].wind_direction_deg); } else { Serial.printf("Wind max: [--.-m/s] Wind avg: [--.-m/s] Wind dir: [---.-deg] "); } if (weatherSensor.sensor[i].rain_ok) { Serial.printf("Rain: [%7.1fmm] ", weatherSensor.sensor[i].rain_mm); amms.setSensorField("precipitation", weatherSensor.sensor[i].rain_mm); } else { Serial.printf("Rain: [-----.-mm] "); } if (weatherSensor.sensor[i].moisture_ok) { Serial.printf("Moisture: [%2d%%] ", weatherSensor.sensor[i].moisture); } else { Serial.printf("Moisture: [--%%] "); } Serial.printf("RSSI: [%5.1fdBm]\n", weatherSensor.sensor[i].rssi); Serial.println("Sending data"); amms.sendData(); } delay(100); }
Explanation of the code
The only configuration parameter to be set in the programme is the token of the station that allows the system to authenticate itself with the AMMS server:
String AMMS_TOKEN = 'STATION_TOKEN_HERE';
When the ESP32 starts, the programme initialises the radio module
weatherSensor.begin();
and tries to connect to the Wi-Fi network. If no network is saved, it will make the Wi-Fi network available Bresser Gateway which will allow the user to configure the parameters for the Internet connection.
wifiManager.autoConnect('Bresser Gateway');
Once connected to the Internet, the gateway listens for new incoming messages from the radio module.
int decode_status = weatherSensor.getMessage();
Upon receiving a new message, it proceeds to print the content in the serial
Serial.printf("Temp: [%5.1fC] ", weatherSensor.sensor[i].temp_c);
and adding sensor data to the sending queue of the AMMS server.
amms.setSensorField("temperature", weatherSensor.sensor[i].temp_c);
Once the collection and printing cycle is finished, it proceeds with sending the data to the server.
amms.sendData();
The Bresser Gateway can be easily configured via the web interface that is available at first start-up. To reach the configuration page, simply connect to the Wi-Fi network Bresser GatewayOnce connected, you will be automatically redirected to the configuration page, where you can enter the credentials of the Wi-Fi network to which you intend to connect the device.
Conclusions
Having reached this point, the data acquired by the Bresser became 'interpretable' with all the most common systems, from sending it to platforms such as Thingspeak or Arduino cloud to operating actuators driven by weather parameters (e.g, a relay that activates at certain temperatures) to creating a display with info from multiple stations (the device reads all the Bressers detected in the reception range of the antenna, each assigned to its own receiver ID in the original display itself), so it is possible to exploit the system for more than infinite uses, the only limitation being imagination
The system, which is very cheap (70€ for the station and 40€ for the hardware including ESP, RF module and cables), is very compact, and allows even a fairly closed station to be made OpenSource, enabling data to be shared with larger projects, for other makers in the form of OpenData, and above all, it can be used at will
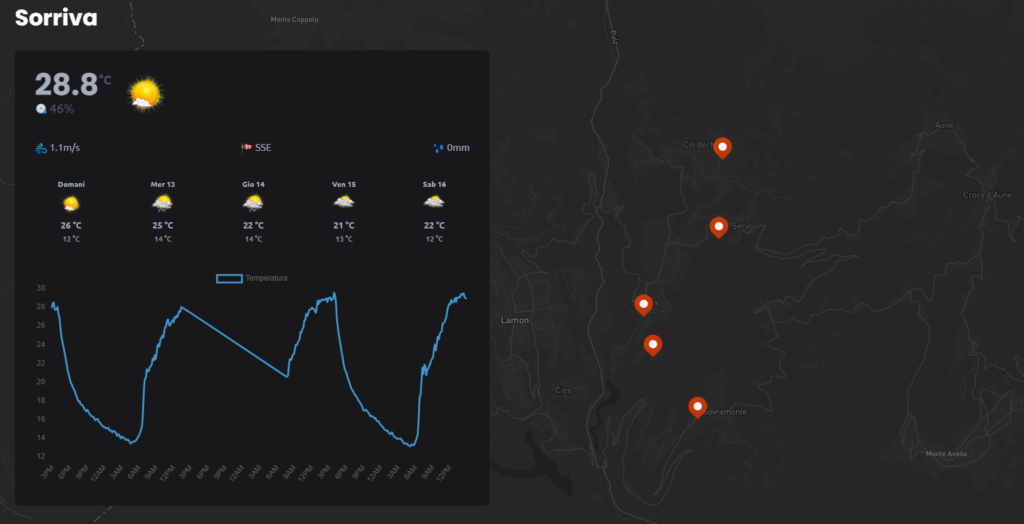
Future improvements
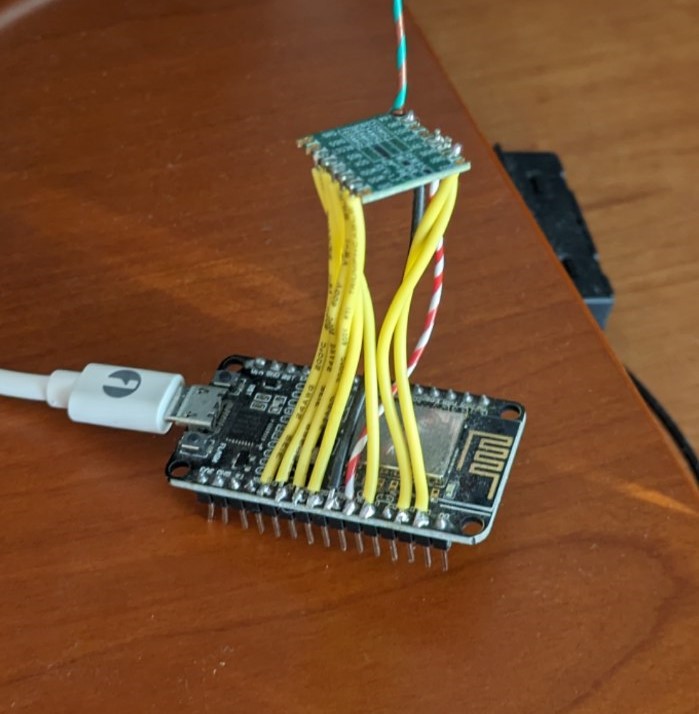
The system lends itself to endless customisation and consequent improvements.
The first of these is to use the dev board of your choice, the important thing being that it is an ESP32 and that the connection pins are respected
Subsequently, we are thinking of building a dedicated PCB for the system, in order to make it easier to build the prototype for sending data, as well as allowing more hardware outputs to connect batteries, displays, actuators and more
Do you have ideas and proposals for improving the project? In addition to the GitHub of the project you can contact us in the group Telegram where you can talk directly to the developers!
Glossary
In this section you will find the definitions of the words in bold in the article:
- AMMS: AUTONOMOUS METEREOLOGICAL MONITORING SYSTEM, is the weather system created by Klinkon Electronics in collaboration with the DeLucaLabs monitoring the environment where the control units are located and providing free data to all
- OpenData: data freely accessible to all whose possible restrictions are the obligation to cite the source or to keep the database open at all times
- 868 MHzThese are ISM (Industry, Science and Medicine) radio frequency bands. These ISM bands are used in various small to large public or industrial applications where range, cost and range criteria are critical.
- Gatewayin computer science and telecommunications, and in particular in computer networks, is a network device that connects two computer networks of different types.
- IoTThis refers to the process of connecting everyday physical objects to the Internet. IoT devices embedded in these physical objects mainly fall into one of these two categories: switches (which send a command to an object) or sensors (which acquire data and send it elsewhere).
- OpenSourceAn open source licence means that the authors, instead of prohibiting, allow not only to use and copy, but also to modify, extend, elaborate, sell and whatever else, all this without imposing obligations to financially reward the authors, a given project being in a well-defined repository.
- Forkmeans the development of a new software project from the source code of an already existing one, by a programmer
- Token: a unique indicator recorded in an access register, with the function of representing a digital object, certifying the ownership of an asset or allowing access to a service, in this case access to our data upload service.
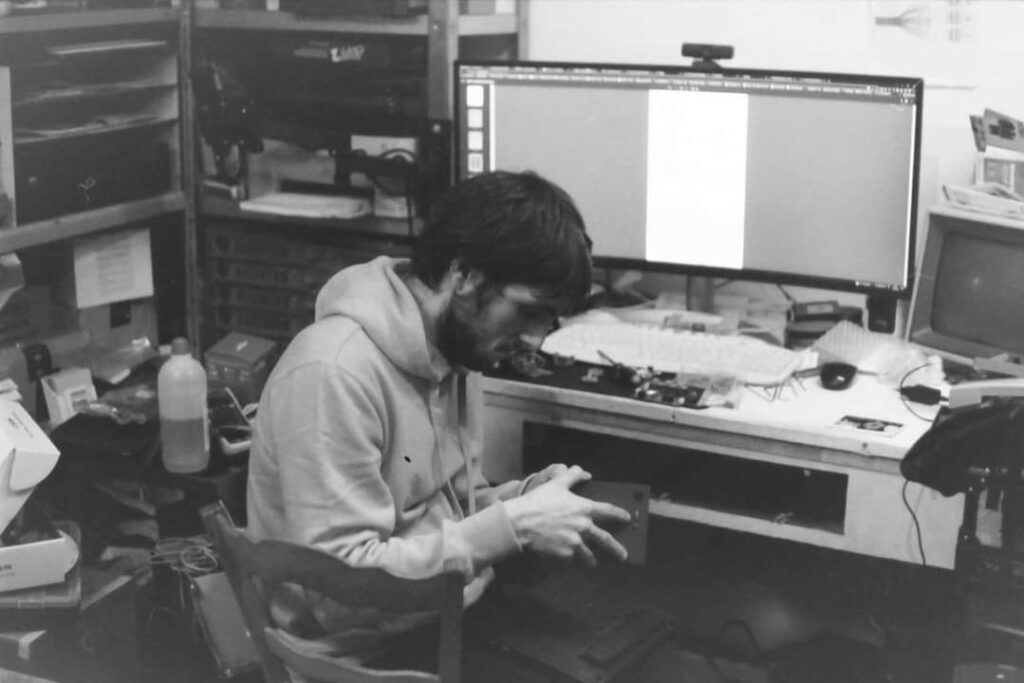
Giosuè is a computer science student with a passion for electronics and servers. Specialised in the development of WEB solutions, Giosuè is the mastermind of all our programmes and takes care of the computer systems of our weather system, as well as the weather stations and data collection server, and works full-time with both us and DeLucaLabs
2 Responses
I have read and understood everything that I could understand.Excellent idea and also economical.Too bad that the good Joshua has already put his hand to my station and solved problems that were insurmountable for me.I hope that this project of yours will become a mast for many of us...but I think it needs to be publicised as well as possible.Good work.
Thank you very much Biagio!
The idea is precisely to make it as simple and inexpensive as possible to convert all commercial weather stations from Amazon, such as the aforementioned Bresser stations that transmit data from station to control unit via an 868Mhz frequency. It is no coincidence that we are currently working on simplifying things even further by releasing a PCB where all you have to do is solder the components in the right position and load the firmware we released to have all the data available